We have recently been working with a client on their cookie compliance. After performing an audit across all their sites, the question was raised about whether we could track in Adobe Analytics which cookies are being fired and then trigger an alert when new cookies appear.
It took a surprisingly small amount of code to capture this information and the reports are interesting.
Capturing the cookie names
Cookies are stored in the DOM in object called document.cookie
. This is a semicolon separated list of name/value pairs, holding the names and values of the cookies. We don’t want to capture the values of the cookies as they are generally too long for an Adobe list var, they may (but shouldn’t!) contain personally identifiable information (or anonymised user IDs at least) and the different values will cause duplication in the reports.
So the first thing the JS needs to do is separate the full list into individual cookies:
var cookies = document.cookie.split(";");
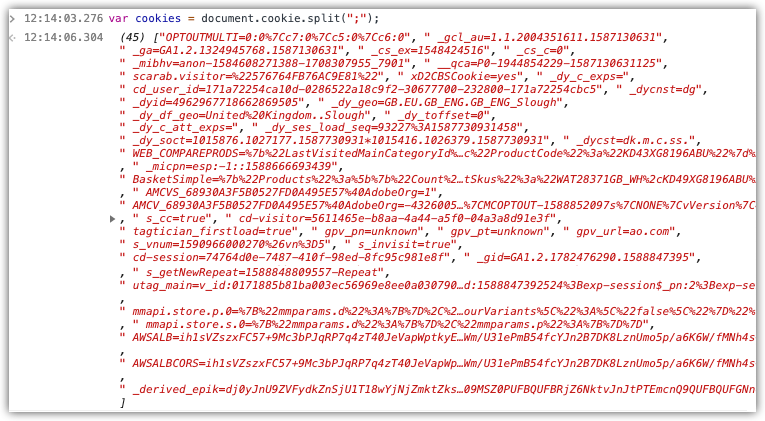
var cookieNames = []; cookies.forEach(function(item){ cookieNames.push(item.split("=")[0].trim()) });
Array.forEach()
method will loop through the items of the array and run the function that is passed in as a parameter. The parameter of the function is a reference to the array item in the loop.cookieNames
.cookies.forEach((item) => { cookieNames.push(item.split("=")[0].trim()) });
Finally we need to save the array into a delimited string to match the configuration in Adobe:
s.list3 = cookieNames.join(",");
The character used in the join()
method should match the delimiter character configured in the appropriate list var.
The expiry is set to Hit as we don’t want this information to persist. We’re only interested in the number of instances/occurrences of each cookie name.
You can then do a trended report of this list var. In most cases this will give a whole load of roughly parallel lines, as most people will get all the cookies (unless they have opted out of particular categories). The interest comes when you see a line start from 0, indicating a new cookie, or a line disappear to 0, indicating a cookie that is no longer present.
You could also add a metric to capture the number of cookies on a page, which would just be the length of the array that you assigned to list var 3. If you capture another counter Event at the same time, you can use that as an Instance counter, which you can then use to get an average number of cookies per hit (or you could use page views).
s.list3 = cookieNames.join(","); s.events = "event100=" + cookieNames.length + ",event101";
You can then add an alert on this average number of cookies metric, so you’ll get an email if the number changes.
Ben Stephenson has worked as a consultant for 120Feet for four years. Before 120Feet he had ten years of development experience on ecommerce websites, and five years of digital marketing experience as an ecommerce manager looking after a large multinational collection of websites for a Travel and Tourism company. He is an official Tealium Expert (with the polo shirt to prove it!) and spends most of his days in Adobe Analytics, Tealium iQ, and Adobe Launch.
In his spare time he plays guitar, builds Lego and takes photos.